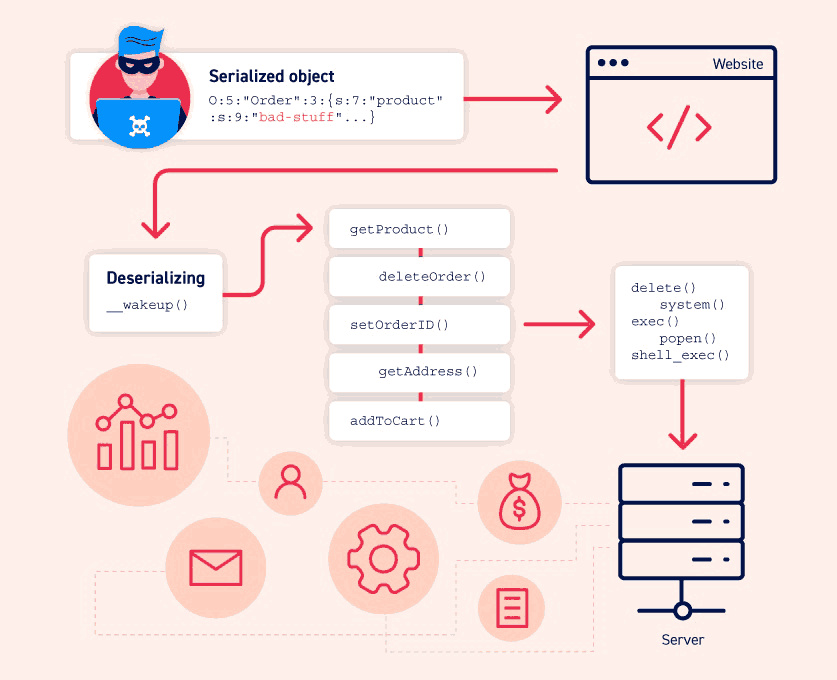
In the world of PHP development, serialize()
and unserialize()
are indispensable functions. They allow us to transform complex PHP data structures – arrays, objects, and scalar values – into a storable string representation and then reconstruct them perfectly. This is incredibly useful for tasks like storing data in databases, managing sessions, or passing information between different parts of an application.
However, this seemingly seamless process can be fraught with peril. A subtle but significant issue can lead to the silent corruption of your serialized data, causing headaches and unexpected errors when you try to bring it back to life with unserialize()
. The culprits? Common characters like double quotes ("
), apostrophes ('
), colons (:
), and semicolons (;
) lurking within the data being serialized.
The Anatomy of Data Corruption
The problem arises when these specific characters, which also happen to be structural elements within the serialized string format itself, are not handled correctly during the storage process, particularly when saving directly into a database using standard SQL queries.
Consider this seemingly innocent PHP array:
$data = array( "hello", "world", "It's a test"); $saving_data = serialize( $data ); echo $saving_data; // Output might look something like: a:3:{i:0;s:5:"hello";i:1;s:5:"world";i:2;s:9:"It's a test";}
Now, imagine trying to save this directly into a database field using a simple SQL UPDATE statement:
$sql_query = "UPDATE your_table SET data = '" . $saving_data . "' WHERE id = 1"; // Potential issue here!
The issue lies in how the database and the SQL query interpret the single quote ('
) within the string “It’s a test”. The database might prematurely terminate the string value being inserted, leading to an incomplete or altered serialized string being stored. When you later retrieve this corrupted string and attempt to unserialize()
it, PHP will encounter an unexpected format, resulting in errors or a failure to reconstruct the original data. You might see warnings, errors, or simply get false or null as the result of unserialize()
.
The Base64 Guardian: A Safe Passage for Serialized Data
Fortunately, there’s a robust and straightforward technique to safeguard your serialized data during storage and retrieval: leveraging base64_encode()
and base64_decode()
.
Here’s the strategy:
Safe Serialization: Before saving your serialized data, encode it using base64_encode():
$value_to_store = array( "This has \"quotes\"", "and 'apostrophes'", "also: colons;", "and finally; semicolons." ); $safe_to_store = base64_encode(serialize($value_to_store)); // Now $safe_to_store contains a string safe for database storage.
base64_encode()
converts the binary representation of the serialized string into an ASCII string using a limited set of characters, effectively neutralizing the problematic characters that could confuse the database.
Faithful Unserialization: When retrieving the data from the database, first decode it using base64_decode()
before unserializing:
$fetched_from_db = "... (your database retrieval logic here) ..."; // Contains the base64 encoded string. $original_value = unserialize(base64_decode($fetched_from_db)); // $original_value now holds the original PHP data structure, intact.
base64_decode()
reverses the encoding process, giving you back the original serialized string, which unserialize()
can then process correctly.
Why This Works
Base64 encoding transforms data into a format composed of a limited set of ASCII characters (A-Z, a-z, 0-9, +, /, and =). This encoding effectively masks the special characters that have structural meaning in serialized PHP strings, preventing the database from misinterpreting them during the save operation. When you decode the base64 string upon retrieval, you reconstruct the exact serialized representation that PHP expects for unserialize()
to function flawlessly.
A Word of Caution: Database Abstraction Layers
While the direct SQL example highlights the core issue, it’s important to note that if you’re using a robust database abstraction layer like PDO in PHP or WordPress’s built-in database functions ($wpdb
), these systems often handle the proper escaping and quoting of data for you, mitigating the risk of corruption.
For instance, in WordPress, using $wpdb->insert()
or $wpdb->update()
with prepared statements ensures that data is safely inserted into the database without the need for manual escaping that can sometimes go wrong with serialized strings.
Conclusion
The power of serialize()
and unserialize()
comes with a caveat: the potential for data corruption during storage if special characters aren’t handled correctly. By adopting the simple yet effective strategy of using base64_encode()
before saving and base64_decode()
after retrieving serialized data, you can build more robust and reliable PHP applications, ensuring that your valuable data remains intact throughout its lifecycle. While modern database abstraction layers offer significant protection, understanding the underlying issue and having the base64 encoding technique in your toolkit provides an extra layer of security and peace of mind.